Summary: in this tutorial, you’ll learn how to stop a thread in Python from the main thread using the Event class of the threading module.
Introduction to the Event object
To stop a thread, you use the Event
class of the threading module. The Event
class has an internal thread-safe boolean flag that can be set to True
or False
. By default, the internal flag is False
.
In the Event
class, the set()
method sets the internal flag to True
while the clear()
method resets the flag to False
. Also, the is_set()
method returns True
if the internal flag is set to True
.
To stop a child thread from the main thread, you use an Event
object with the following steps:
- First, create a new
Event
object and pass it to a child thread. - Second, periodically check if the internal flag of the
Event
object is set in the child thread by calling theis_set()
method and stop the child thread if the internal flag was set. - Third, call the
set()
method in the main thread at some point in time to stop the child thread.
The following flow chart illustrates the above steps:
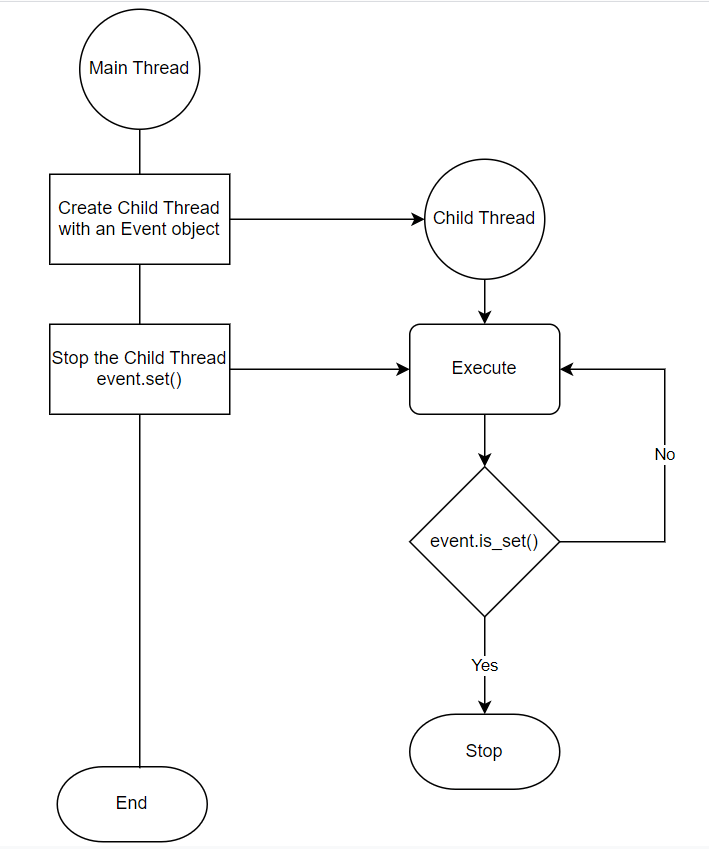
A simple example of stopping a thread in Python
The following example shows how to use an Event
object to stop a child thread from the main thread:
from threading import Thread, Event from time import sleep def task(event: Event) -> None: for i in range(6): print(f'Running #{i+1}') sleep(1) if event.is_set(): print('The thread was stopped prematurely.') break else: print('The thread was stopped maturely.') def main() -> None: event = Event() thread = Thread(target=task, args=(event,)) # start the thread thread.start() # suspend the thread after 3 seconds sleep(3) # stop the child thread event.set() if __name__ == '__main__': main()
Code language: Python (python)
The task() function
The task()
function uses an Event
object and returns None
. It will be executed in a child thread:
def task(event: Event) -> None: for i in range(6): print(f'Running #{i+1}') sleep(1) if event.is_set(): print('The thread was stopped prematurely.') break else: print('The thread was stopped maturely.')
Code language: Python (python)
The task()
function iterates over the numbers from 1 to 5. In each iteration, we use the sleep()
function to delay the execution and exit the loop if the internal flag of the event object is set.
The main() function
First, create a new Event
object:
event = Event()
Code language: Python (python)
Next, create a new thread that executes the task()
function with an argument as the Event
object:
thread = Thread(target=task, args=(event,))
Code language: Python (python)
Then, start executing the child thread:
thread.start()
Code language: Python (python)
After that, suspend the main thread for three seconds:
sleep(3)
Code language: Python (python)
Finally, set the internal flag of the Event object to True by calling the set()
method. This will also stop the child thread:
event.set()
Code language: Python (python)
Stopping a thread that uses a child class of the Thread class
Sometimes, you may want to extend the Thread
class and override the run()
method for creating a new thread:
class MyThread(Thread): def run(self): pass
Code language: Python (python)
To stop the thread that uses a derived class of the Thread
class, you also use the Event
object of the threading module.
The following example shows how to create a child thread using a derived class of the Thread
class and uses the Event
object to stop the child thread from the main thread in demand:
from threading import Thread, Event from time import sleep class Worker(Thread): def __init__(self, event, *args, **kwargs): super().__init__(*args, **kwargs) self.event = event def run(self) -> None: for i in range(6): print(f'Running #{i+1}') sleep(1) if self.event.is_set(): print('The thread was stopped prematurely.') break else: print('The thread was stopped maturely.') def main() -> None: # create a new Event object event = Event() # create a new Worker thread thread = Worker(event) # start the thread thread.start() # suspend the thread after 3 seconds sleep(3) # stop the child thread event.set() if __name__ == '__main__': main()
Code language: Python (python)
How it works.
First, define a Worker
class that extends the Thread
class from the threading module. The __init__()
method of the Worker
class accepts an Event
object.
Second, override the run()
method of the Worker
class and use the event object to stop the thread.
Third, define the main()
function that creates an event, a Worker
thread, and passes the event object to the Worker
thread. The remaining logic is the same as the main()
function in the previous example.
Leave a Reply