In the multi-page example above (see Listing 2–3) we saw how jQuery Mobile navigates from one internal page to another. When the multi-page document was initialized, both internal pages were already added to the DOM so the page transition from one internal page to the other was extremely fast. When navigating from page to page we can configure the type of transition to apply. By default, the framework will apply a "slide"
effect for all transitions. We will discuss transitions and the types of transitions we can choose from later in the chapter.
<!-- Navigate to an internal page -->
<div data-role="content">
<a href="#contact" data-role="button">Contact Us</a>
</div>
The navigation model is different when a single-page transitions to another single-page. For instance, we could extract the contact page from our multi-page into its own file (contact.html
). Now our home page (hijax.html
) can access the contact page as a normal HTTP link reference:
Listing 2–4. Ajax Navigation (ch2/hijax.html
)<div data-role="content">
<a href="contact.html" data-role="button">Contact Us</a>
</div>
When clicking the “Contact Us” link above, jQuery Mobile will process that request as follows:
- jQuery Mobile will parse the
href
and load the page via an Ajax request (Hijax). For a visual, refer to Figure 2–3. If the page is loaded successfully, it will be added to the DOM of the current page.Figure 2–3. jQuery Mobile Hijax RequestWith the page successfully added to the DOM, jQuery Mobile will enhance the page as necessary, update the
base
element’s@href
, and set thedata-url
attribute if it was not explicitly set. - The framework will then transition to the new page with the default “
slide
” transition applied. The framework is able to achieve a seamless CSS transition because both the “from” and “to” pages exist together in the DOM. After the transition is complete, the page that is currently visible or active will be assigned the “ui-page-active” CSS class. - The resulting URL is also bookmarkable. For example, if you want to deep link to the contact page you may access it from its full path:
http://<host:port>/ch2/contact.html.
NOTE: As an added bonus, Ajax-based navigation will also produce clean URLs in browsers that support HTML5’s pushState. This feature is supported in the recent versions of desktop Safari, Chrome, Firefox, and Opera. Android (2.2+) and iOS5 also support pushState. In browsers that do not support this feature, the hash-based URLs (http://<host:port>/hijax.html#contact.html
) will be used to preserve the ability to share and bookmark URLs. - If any page fails to load within jQuery Mobile, a small error message overlay of “Error Loading Page” will be shown and faded out (see Figure 2–4).
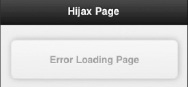
Figure 2–4. Error Loading Screen
$.mobile.changePage()
- The changePage function handles all the details of transitioning from one page to another. You are allowed to transition to any page except the same page. The complete list of available transition types are shown in Table 2–1.
Usage
- $.mobile.changePage( toPage, [options] )
Arguments
toPage
(string or jQuery collection). The page to transition to.toPage
(string). A file URL ("contact.html"
) or internal element’s ID (“#contact
”).toPage
(jQuery collection). A jQuery collection containing a page element as its first argument.
options
(object). A set of key/value pairs that configure the changePage request. All settings are optional.transition
(string, default: $.mobile.defaultTransition). The transition to apply for the change page. The default transition is"slide"
.reverse
(boolean, default:false
). To indicate if the transition should go forward or reverse. The default transition is forward.changeHash
(boolean, default:true
). Update the hash to the page’s URL when page change is complete.role
(string, default:"page"
). The data-role value to be used when displaying the page. For dialogs use"dialog"
.pageContainer
(jQuery collection, default:$.mobile.pageContainer
). Specifies the element that should contain the page after it is loaded.type
(string, default:"get"
). Specifies the method (“get
” or “post
”) to use when making a page request.data
(string or object, default: undefined). The data to send to an Ajax page request.reloadPage
(boolean, default:false
). Force a reload of the page, even if it is already in the DOM of the page container.showLoadMsg
(boolean, default:true
). Display the loading message when a page is requested.fromHashChange
(boolean, default:false
). To indicate if the changePage came from a hashchange event.
Example #1:
//Transition to the "contact.html" page.
$.mobile.changePage( "contact.html" );
<!-- Markup equivalent -->
<a href="contact.html">Contact Us</a>
Example #2:
// Go to an internal "#contact" page with a reverse "pop" transition.
$.mobile.changePage( '#contact', { transition: "pop", reverse: true } );
<!-- Markup equivalent -->
<a href="contact.html" data-transition="pop" data-direction="reverse">
Contact
</a>
Example #3:
/* Dynamically create a new page and open it */
// Create page markup
var newPage = $("<div data-role=page data-url=hi><div data-role=header>
<h1>Hi</h1></div><div data-role=content>Hello Again!</div></div>");
// Add page to page container
newPage.appendTo( $.mobile.pageContainer );
// Enhance and open new page
$.mobile.changePage( newPage );
IMPORTANT: Ajax navigation will not be used for situations where you load an external link:
<!-- Ajax navigation will be ignored when loading a page with a
rel="external" or target attribute -->
<a href="multi-page.html" rel="external">Home</a>
<!-- Ajax navigation will be ignored -->
<a href="multi-page.html" target="_blank">Home</a>
Under these conditions, normal HTTP request processing will occur. Furthermore, CSS transitions will not be applied. As mentioned earlier, the framework is able to achieve smooth transitions by dynamically loading the “from” and “to” pages into the same DOM and then applying a smooth CSS transition. Without Ajax navigation the transition will not appear as smooth and the default loading message ($.mobile.loadingMessage
) will not be shown during the transition.
Configuring Ajax Navigation
Ajax navigation is enabled globally but you can disable this feature if DOM size is a concern or if you need to support a particular device that does not support hash history updates (see Note below). By default, jQuery Mobile will manage the DOM size or cache for us with only the “from” and “to” pages involved in the active page transition merged into the DOM. To disable Ajax navigation set $.mobile.ajaxEnabled = false when binding to the mobileinit event. For further information about configuring jQuery Mobile or managing the DOM cache refer to Chapter 8.
NOTE: Ajax navigation has been disabled on platforms that have known conflicts with hash history updates. For instance, jQuery Mobile has disabled Ajax navigation ($.mobile.ajaxEnabled = false)
for BlackBerry 5, Opera Mini (5.0-6.0), Nokia Symbian^3, and Windows Phone 6.5. These devices are more usable when browsing with regular HTTP and full-page refreshes.CopycopyHighlighthighlightAdd NotenoteGet Linklink
Leave a Reply