In our prior example we saw how to POST form data to the server. In this example, we will use a GET request to fetch data from the server. This example will retrieve a listing of movies from the server and display the results within a jQuery Mobile JSP page (see Figure 9–7).
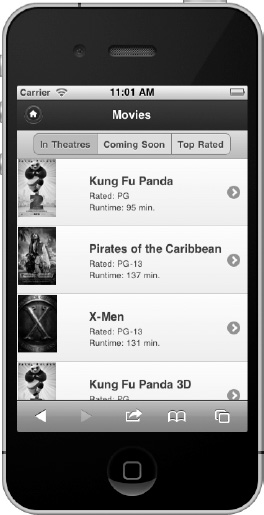
Figure 9–7. Movies fetched from server-side MVC access
On the server-side, we have a Spring MVC controller setup to handle GET requests on the following href:
<a href="/jqm-webapp/mvc/movies" data-role=”button”>Movies</a>
When the button is clicked, a GET request will be triggered and sent to our MoviesController. The MoviesController will retrieve our movie data and forward the response to the movies JSP page (see Listing 9–15).
Listing 9–15. MVC Controller to GET movie data (com.bmb.jqm.MoviesController.java)@Controller()
public class MoviesController {
@RequestMapping(method = RequestMethod.GET)
public String getMovies(ModelMap model) {
model.addAttribute("movies", getMovieData());
return "movies";
}
}
The response will be forwarded to the movies page where the JSP will iterate the list of movies displaying a separate list item for each result (see Listing 9–16).
Listing 9–16. JSP to display movie data (/jsp/movies.jsp)<div data-role="content">
<ul data-role="listview">
<c:forEach var="movie" items="${movies}">
<li>
<a href="#">
<img src="../images/${movie.image}" />
<h3>${movie.title}</h3>
<p>Rated: ${movie.rating}</p>
<p>Runtime: ${movie.runtime} min.</p>
</a>
</li>
</c:forEach>
</ul>
</div>
One advantage of this server-side solution is the simplicity of the page markup. There is no dynamic page generation with JavaScript, string concatenation, or dynamic field binding with jQuery selectors that we saw earlier in our client-side examples.
Leave a Reply