The source files are simple text files. Having a useful programming editor with language support is as convenient for COBOL as any other language, if not more so. The easiest thing for a beginner is to use Visual Studio Code, the only competitor for my affections since EMACS.
There are surprisingly many VSCode extensions for COBOL. Right now, I’m using the bitlang code colorizer and Broadcom COBOL language support. A lot of the others are intended for people programming in a mainframe environment, but that adds complexity we don’t need for an introduction.
So, to summarize, to begin to experiment with COBOL:
- Download and install Visual Studio Code if you haven’t already.
- Install the bitlang.cobol and Broadcom COBOL Language Support extensions.
- Install GnuCOBOL. (Honestly, if anything is going to cause trouble, it will be this. The Homebrew installation on MacOS worked fine, and I don’t have other systems with which to test. On Windows, MicroFocus has a free trial for Visual Studio COBOL and Azure support for experimentation.)
There you are, you’ve installed everything and you’re ready to write your first COBOL program. As is traditional, we’ll start with the Ur-program, “Hello, world”.
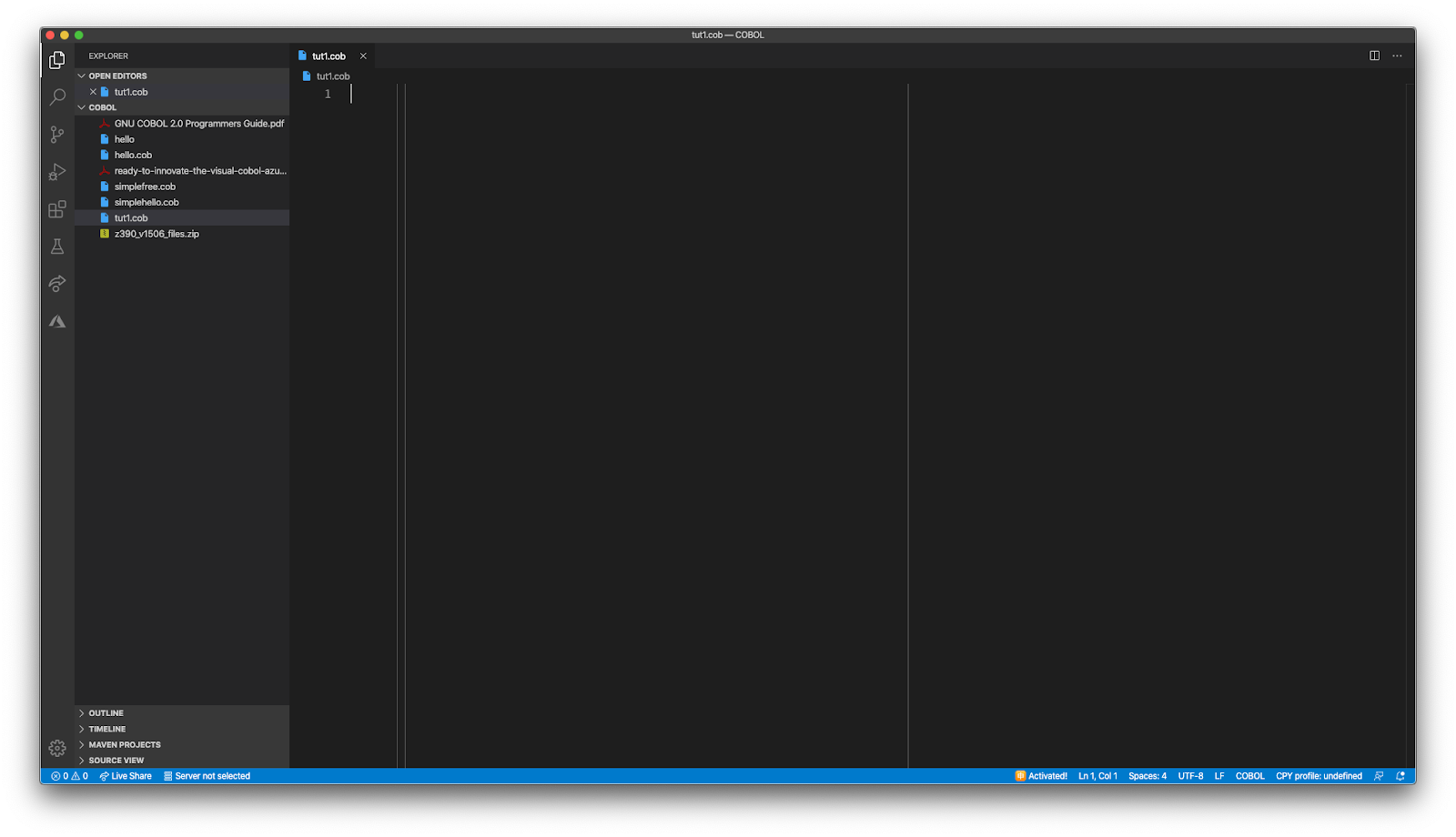
So here’s your first surprise as a new COBOL programmer: COBOL cares about what column your code is in. In a traditional COBOL program, the source has several components:
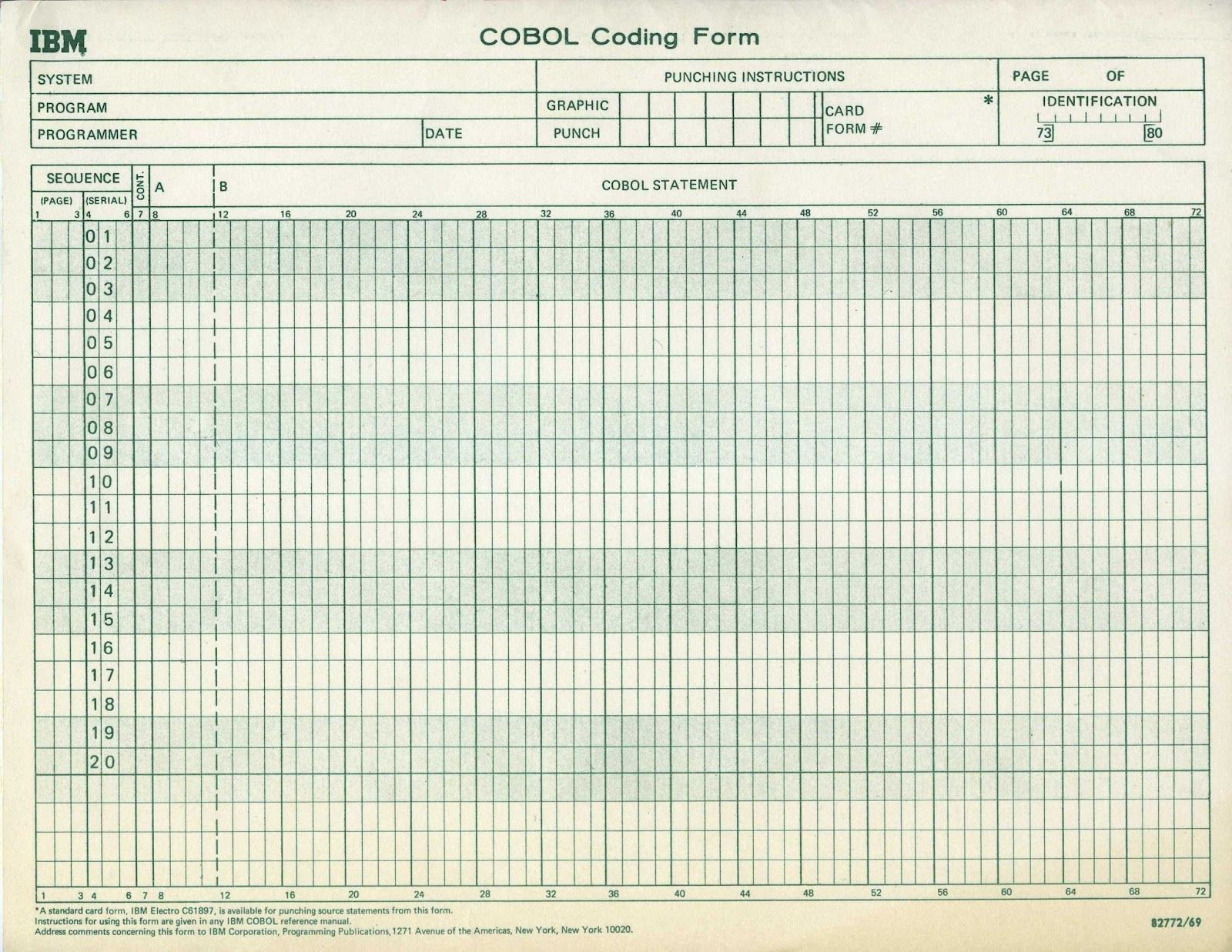
Columns 1-6 are there for a sequence number. Column 7 is called the indicator area; in general, it’s mostly used to indicate comments by putting an asterisk ‘*’ in that column. Code then goes in columns 8 through 72, and columns 73-80 are basically free for the programmers use.
This is all based around the days when we put our source into 80-column Hollerith cards.
Modern COBOL compilers also accept a free format which doesn’t force your code into the 80-column straitjacket, but a very large proportion of existing code is still in the card-image format. For right now, we’ll stick with card images.
Brace yourselves: COBOL is not a block-structured language like nearly any other language you’ve ever used. A major design goal for COBOL from the first was that it should be “self-documenting” with an English-like syntax. Instead of having functions or subroutines and blocks, we have divisions, sections, paragraphs, and statements. (We’ll see something almost like a subroutine with the PERFORM verb below.)
Oh, right, we also have verbs for COBOL operators.
Here’s “Hello, World” in COBOL:
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO.
PROCEDURE DIVISION.
DISPLAY "Hello, world".
END PROGRAM HELLO.
Compared to some languages it’s a little wordy, but honestly not so bad. Compare it to a simple Java version:
public class Hello {
public static void main(String[] args){
System.out.println("Hello, world!");
}
}
Like all “Hello, world” programs it doesn’t do much—but if you’ve been told that it takes 90 lines to write a basic program in COBOL, well, you’ve been misled.
Now let’s take the “Hello world” program apart for our first example.
The first line is:
IDENTIFICATION DIVISION.
COBOL programs always have at least an identification division and a procedure division. The identification division has one important paragraph, the PROGRAM-ID. You need to give the program a name here. The name doesn’t need to correspond to the file name or pretty much anything, except when your COBOL program is being called from another COBOL program. This is through the CALL verb, which we’re not going to cover.
We do need to have a program ID, so we add
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO.
There are a lot of other things that commonly go into the identification division. I’ll add a couple of common examples.
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO.
AUTHOR. CHARLES R MARTIN.
DATE-WRITTEN. 2020-APR-11.
In modern environments, however, these are comments.
Speaking of modern environments, by the way, COBOL doesn’t require all-caps like I’ve been using. GnuCOBOL would be perfectly happy with
identification division.
program-id. tut2.
author. charlie martin.
procedure division.
display "hello, world".
end program tut2.
I’m just having a little misty-eyed nostalgia here.
Don’t judge me.So let’s finish up our “Hello, world.” The execution part of a COBOL program is in the procedure division.
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO.
PROCEDURE DIVISION.
DISPLAY "Hello, world".
END PROGRAM HELLO.
There’s one more bit of card-image format here. Notice that `DISPLAY “Hello, world”` is indented four columns. That’s because the part from column 8-72 actually has two parts: the A section, from column 8-11, and the B section from column 12 on. Divisions, sections, and paragraphs need to start in the A section; code statements should start in the B section.
Leave a Reply