Npm is the package that has registered jquery in it. You can install these npm packages by using the npm command in the CLI.
npm install jquery
You can also use yarn if you do not want to use the npm package installer.
yarn add jquery
Using the above command, you ensure that jquery is installed in the node_modules directory. The uncompressed release, a map file, and a compressed release will also be built and found inside node_modules/jquery/dist.
There is an additional way to install jquery. By using Bower, you can list it as a package within Bower and install it through the CLI.
Bower install jquery
The above-listed command will install jquery to the Bower’s install directory. The default path for this directory is bower_components. To find the uncompressed map file and the compressed file, you need to locate them inside the bower_components/jquery/dist. This package contains additional files besides the default distribution package.
You can also directly install the compressed file by writing the command :
Bower install https://code.jquery.com/jquery-3.3.1-min.js
You can also use Jquery CDN if you do not wish to download and host the jquery file yourself and wish to make use of CDN. Both Microsoft and Google host jquery.
Microsoft CDN:
<head>
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.3.1.min.js"></script>
</head>
Google CDN:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery-3.3.1.min.js"></script>
</head>
You can include the Jquery library inside the HTML scripts:
<html>
<head>
<title>Jquery first test example</title>
<script type = "text/javascript" src = "https://cdn.educba.com/jquery/jquery-2.1.3.min.js">
</script>
<script type = "text/javascript">
$(document).ready(function() {
document.write("HI there!");
});
</script>
</head>
<body>
<h1>Hello there</h1>
</body>
</html>
This above step is part of the local installation where we have put the jquery file inside the website’s directory.
Now, we will be looking at the CDN(Content Delivery Network) way of calling jquery:
<html>
<head>
<title>My jquery test sample script</title>
<script type = "text/javascript"
src = "https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type = "text/javascript">
$(document).ready(function() {
document.write("Hello there ");
});
</script>
</head>
<body>
<h1>Hi there</h1>
</body>
</html>
Call jquery library functions:
Almost everything is only done when jquery reads, writes, or manipulates the document object model (DOM) list of elements. When our DOM file is ready, we must ensure that the events and other basic components are added. There are two ways again of making your events do their work. One is to call those events from inside the page; therefore, we can use $(document).ready() function. All the content written inside it will be loaded as it is the moment the DOM is loaded and long before the page contents are loaded. You can register a ready event to ensure this happens.
$(document.ready(function) {
// do stuff when DOM is ready
});
When you wish to call further your jquery function, which is a pre-built or a library function, we will use the HTML scripts tag as below.
<html>
<head>
<title>My jquery testing first script</title>
<script type = "text/javascript"
src = "https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type = "text/javascript" language = "javascript">
$(document).ready(function() {
$("div").click(function() {alert("Hello there");});
});
</script>
</head>
<body>
<div id = "mydiv">click me
</div>
</body>
</html>
To further extend the capabilities of jquery scripts, we also have the provision of custom scripts such as custom.
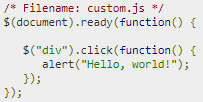
We can extend the capabilities again by calling it inside the HTML tags.
<html>
<head>
<title>My first jquery testing script</title>
<script type = "text/javascript"
src = "https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type = "text/javascript" src = "https://cdn.educba.com/jquery/custom.js">
</script>
</head>
<body>
<div id = "mydiv">
Click me
</div>
</body>
</html>
Jquery also allows multiple libraries all at once without disturbing each other. One other library is MooTool javascript libraries, and jquery together can be used effectively.
Other syntactical things are more relevant for jquery, such as using a $selector.action() field where the $ sign is used to symbolize the query as in access and define it.
You use a selector to query or find the HTML elements, while you use the action() to perform actions on the elements. The document-ready events are also important as they ensure that the scripts or the piece of code do not run well in advance before the document has even finished loading or is in the ready state.
$(document).ready(function(){
//jQuery methods go here...
});
Waiting for the document to load and become ready before working on it is always advisable. Therefore you can also keep your javascript code inside the head section before your body section.
It is very easy to install Jquery and can be done in multiple ways, unlike many other software or programming languages, as it is a scripting language that is just about a library. The main things to learn while using Jquery are the implementation, syntax, and other relevant code information.
Leave a Reply